Description
Vue Injector is an open-source dependency injection library for Vue.js that was developed by SCAND team. The library includes a number of instances that can be used for the implementation of the dependency injection pattern.
Dependencies are services or objects that a class needs to perform its function. DI is a coding pattern in which a class asks for dependencies from external sources rather than creating them itself, and Vue Injector provides the ability to inject dependencies into a component at the moment of its creation. The library can be used for the development of flexible, efficient, and robust applications that will be easier to test and maintain in the future.
There is a detailed description of the installation process of Vue Injector and the ways it can be used for different purposes, including specification of dependencies for Vue.js components, integration of components, injection of properties into components, etc. Read the documentation to learn all the details.
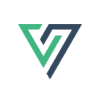
Dependency Injection Vue Key Features
Vue Injector is an open-source dependency injection library for Vue.js that was developed by SCAND team. The library includes a number of instances that can be used for the implementation of the dependency injection pattern. Also, it includes the following:
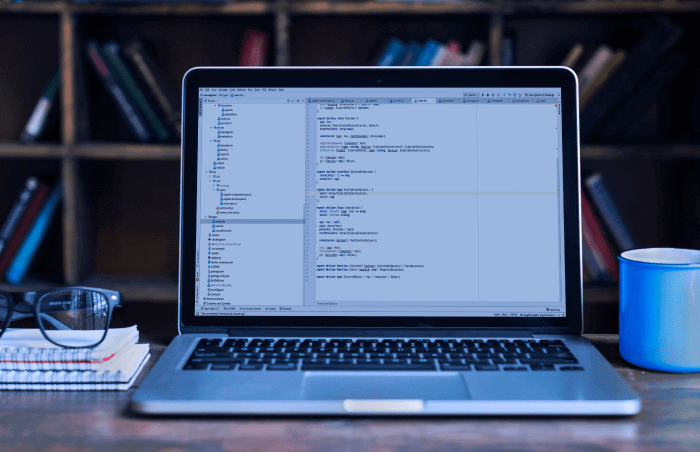
- Dependency injection for components
- Construction of the injected services
- Accessibility of Vue application from service
- Utilization of decorators for convenient operation
Pros of Using Vue Injector
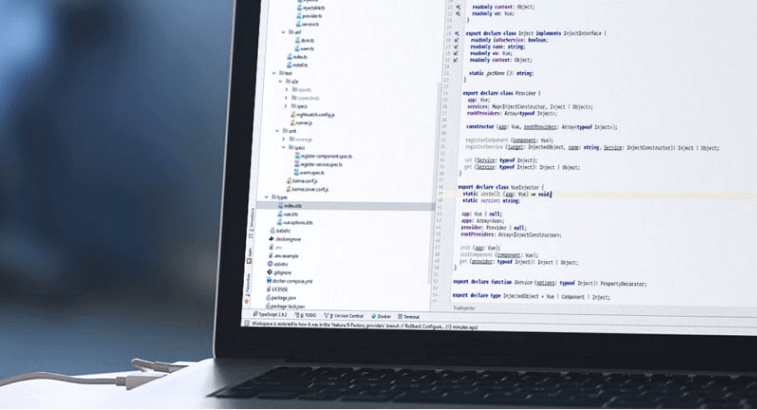
Vue Injector provides some significant benefits that can often be achieved unconsciously, simply by using the initializer-based dependency injection for all objects. The main benefits of the library are the following:
- Provides developers with the ability to utilize dependency injection — an important design pattern that is not included in Vue.js framework by default
- Provides convenient mechanism of data transfer
- Allows creating both a service and a service factory
- Allows requesting the service instead of creating it, providing the ability to depend on interfaces rather than concrete types
- Provides the ability to implement event bus
- Promotes the application’s flexibility, efficiency, and robustness
- Allows creating easily testable and maintainable applications
Vue Injector Code Example
// component/todoList.js /** ... */ /** * You also can use @Inject(Http) httpClient; * It is possible for class-style Vue components. */ import Http from '../services/http'; export default { name: 'TodoList', providers: { httpClient: Http }, created() { this.httpClient .get(URL) /** any pipes */ .subscribe( this.taskHandler ) }, methods: { taskHandler(tasks) { /** ... */ } } } // services/setup.js import Vue from 'vue'; import { VueInjector } from '@scandltd/vue-injector'; Vue.use(VueInjector); export default new VueInjector(); // main.js import injector from './services/setup'; /** ... */ const root = new Vue({ /** ... */ injector }); // services/client.js import axios from 'axios'; import { Injectable } from '@scandltd/vue-injector'; @Injectable({ useFactory: () => axios.create(/** ... */) }) class Client {} export default Client; // services/http.js import { Injectable, Inject } from '@scandltd/vue-injector'; import * as Observable from 'rxjs/internal/observable/fromPromise'; import { map } from 'rxjs/operators'; import Client from './Client'; @Injectable class Http { @Inject(Client) client; observableFactory(promise) { return Observable .fromPromise(promise) .pipe( map(({ data }) => data) ); } /** ... */ get(url, params) { return this.observableFactory( this.client.get(url, { params }) ); } } export default Http
Need a Similar Solution?
Related Products
CurlEffect
CurlEffect is a Xamarin library that enables developers to create slick page turning effects. Easy to use in any app.
epuBear
Lightweight and easily customizable cross-platform EPUB SDK for EPUB readers development.
Realm Browser for Android
Realm Browser Library is a small, but very helpful library designed for viewing and editing Realm database files on Android devices.
ASP.NET MVC Modules
Open source MVC framework for .NET to enable packing multiple resources, including views, icons, scripts, etc. in one DLL.